Wordpress Graphql
For a fun learning & experimentation project, I’ve been building a Dungeons and Dragons character generator. The frontend is built using React JS and deployed to Netlify, and the backend is powered by WordPress and WP GraphQL.
Set-up the API endpoint through the.htaccess file; Instead of defining the API endpoint by code through dependency 'getpop/graphql-endpoint-for-wp' (and having to flush the rewrite rules), it can also be set-up with a rewrite rule in the.htaccess file. For this, remove that dependency from composer, and add the code below before the WordPress rewrite section (which starts with # BEGIN.
I could have tried using a serverless database such as FaunaDB, but I chose WordPress because of familiarity, what it gives you for free, and it’s flexibility when combined with WP GraphQL. I did however need to build a login system. To do this I created a GraphQL login endpoint, and handled user sessions using Cookies.
- We send requests to GraphQL, GraphQL returns data to us and then we do whatever we want with that data. The difference is now that data is generated by WordPress. Now means we can take advantage of all the data creation features in WordPress by creating posts and pages and categories and tags and custom post types and custom fields.
- Posted in Development, GraphQL, Headless, React, WordPress For a fun learning & experimentation project, I’ve been building a Dungeons and Dragons character generator. The frontend is built using React JS and deployed to Netlify, and the backend is powered by WordPress and WP GraphQL.
- It depends on the page being queried. WordPress-GraphQL is designed to cache specific requests and only query specific things when necessary, and resolve and N+1 problems where you would be querying individual things over and over again. WordPress-GraphQL goes through that, but the base design of a WordPress theme in those queries aren't polluting.
Cookies vs JWT
JWT (JSON Web Tokens) can be used to authenticate requests between parties. There is a decent overview of how JWT works here.
A plugin is available for WP GraphQL which handles authentication using JWT and adds a login endpoint. This looked promising, however, the most difficult thing about using JWT in the context of a web based React app is storage of the token. Whilst some articles recommend using localStorage, doing so is not secure—the token could be leaked using XSS.
Don’t store it in local storage (or session storage). If any of the third-party scripts you include in your page gets compromised, it can access all your users’ tokens.
JWT Authentication: When and how to use itTo keep our tokens secure our only option would be to store them in memory. The session would not persist if the browser tab was closed, making it less than ideal.

A better option, and the solution I went with, is using good old fashioned cookies (specifically HTTP Cookies which are inaccessible by JavaScript). I created a new GraphQL route that would set a HTTP cookie after successful login.
Side note: I did find a plugin for WP GraphQL which supports WP cookies, but I encountered browser compatibility (CORS) issues, so ended up rolling my own based on that.
Register a GraphQL Login Mutation
In order to login, we need to register a new endpoint with WP GraphQL. We do this by hooking in to graphql_register_types
and adding our new mutation using the register_graphql_mutation
function documented here.
We’ll call our mutation loginWithCookies
and we’ll use inputFields
to define which fields are accepted (username and password), outputFields
to define the response, and mutateAndGetPayload
to perform the login action:
We can use this mutation using a GraphQL query. You can view my usage of the login mutation in the React app here.

Setting the logged-in cookie
When you log in, WordPress sets cookies to track your session. These cookies have a httponly
parameter so it is not readable through JavaScript.
I found the easiest way to give the headless site access to cookies was to set an additional cookie.After reviewing this, it doesn’t appear to be needed—previously I had issues with Safari but this no longer seems to be a problem. The regular WordPress login cookie is enough.
It doesn’t work yet though, if we try to login we’ll see a CORS error in the browser:
We need to send some additional headers so the browser does not reject the cookie!
Setting CORS Headers
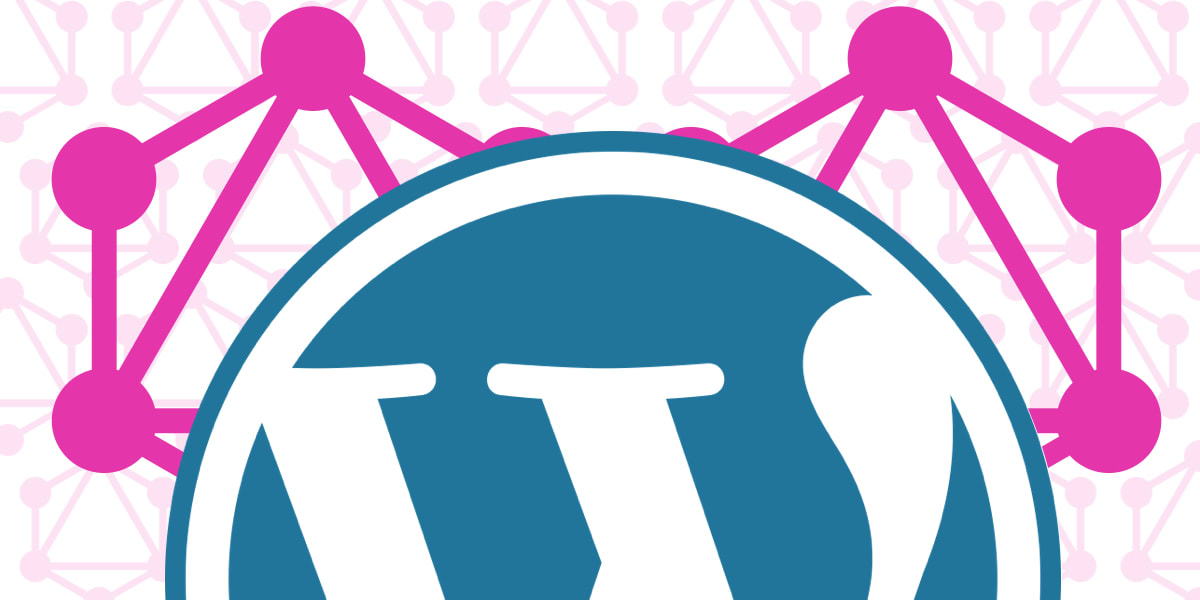
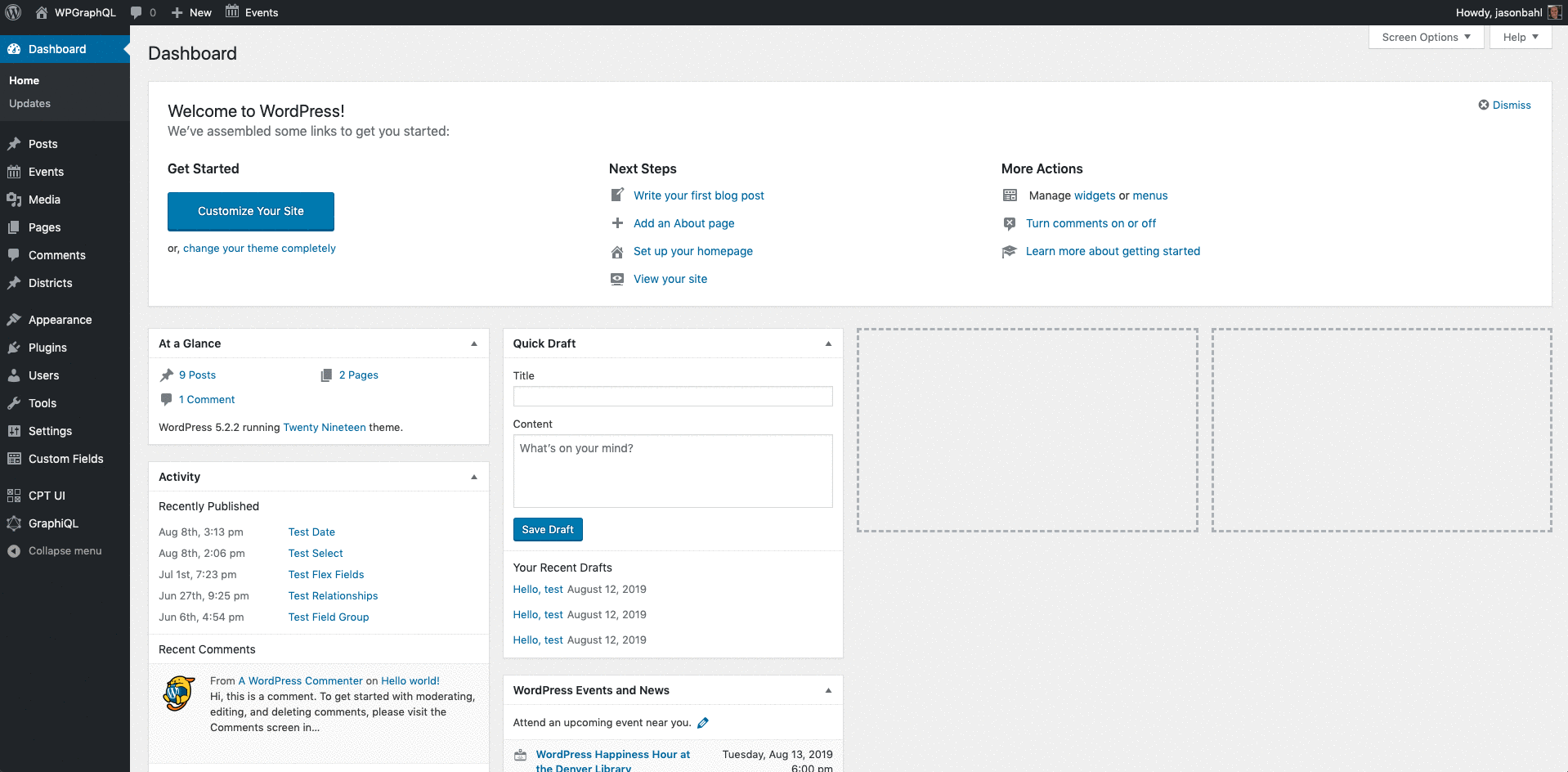
The final piece of the puzzle is telling the browser it should accept the custom cookie when logging in from the headless site. We can define headers for our GraphQL routes using the graphql_response_headers_to_send
hook.
A detailed explanation of CORS can be found in MDN, as well as docs for allow credentials and allow origin.
Wrapping up
We’ve now created a GraphQL mutation which accepts login credentials and logs users in using a custom cookie, and we’ve set CORS headers so the browser allows the cookies to be used. Great! We can now hook this up to a login form in the React app.
Graphql And Wordpress
This is post 1 of 4 in the series “Headless WordPress”
Graphql Api
- Headless WordPress: Cookie Based Login using GraphQL
